Introduction
In the realm of web development, the ability to establish real-time communication between clients and servers is crucial. Enter WebSockets, a powerful technology that opens a bi-directional communication channel over a single TCP connection, unlocking a world of possibilities for web applications. In this article, we will delve into the concepts, practical applications, and best practices of leveraging WebSockets in JavaScript.
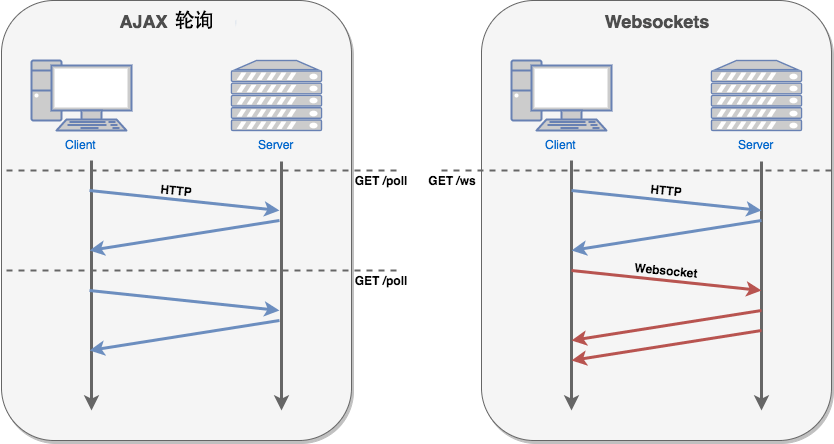
Image: www.runoob.com
Understanding WebSockets
WebSockets are a relatively recent addition to the web technology stack, introduced as a standard in 2011. They provide an upgrade path from traditional HTTP connections, offering significant advantages in terms of scalability, real-time data exchange, and resource efficiency.
At its core, a WebSocket establishes a persistent connection between a client and a server, enabling the exchange of messages back and forth. This connection remains open and active for as long as needed, facilitating real-time communication without the need for constant polling or HTTP requests.
Key Benefits of WebSockets
WebSockets offer several compelling benefits that make them a coveted choice for various types of web applications:
- Real-Time Communication: They allow for instant data exchange between clients and servers, making them ideal for applications that require live updates, such as chat rooms, multiplayer games, and financial dashboards.
- Fast and Efficient: WebSockets leverage binary data for communication, resulting in significantly faster data transfer speeds compared to HTTP requests. This enables applications to handle high-frequency data streams efficiently.
- Persistent Connection: The persistent nature of WebSockets eliminates the need for repeated connection requests. The channel stays open until explicitly closed, reducing latency and improving user experience.
- Low Overhead: WebSockets use a lightweight protocol that minimizes bandwidth usage and overhead. This makes them suitable for applications that handle frequent and small data exchanges.
Practical Applications of WebSockets in JavaScript
The versatility of WebSockets makes them applicable across diverse domains. Here are a few practical examples:
- Chat Applications: WebSockets facilitate real-time messaging in chat applications, allowing users to receive instant updates whenever a new message arrives.
- Multiplayer Games: They enable synchronized and interactive experiences in multiplayer games, where players can communicate and exchange data in real time.
- Financial Trading Platforms: WebSockets provide real-time updates on stock prices, trade executions, and market events, empowering traders with immediate access to critical information.
- Live Event Broadcasting: WebSockets can be employed to stream live events, such as sports games or conferences, delivering real-time updates to viewers.
- Data Monitoring: Applications can monitor sensor data or other real-time information sources using WebSockets, providing a constant stream of data to be analyzed or visualized.
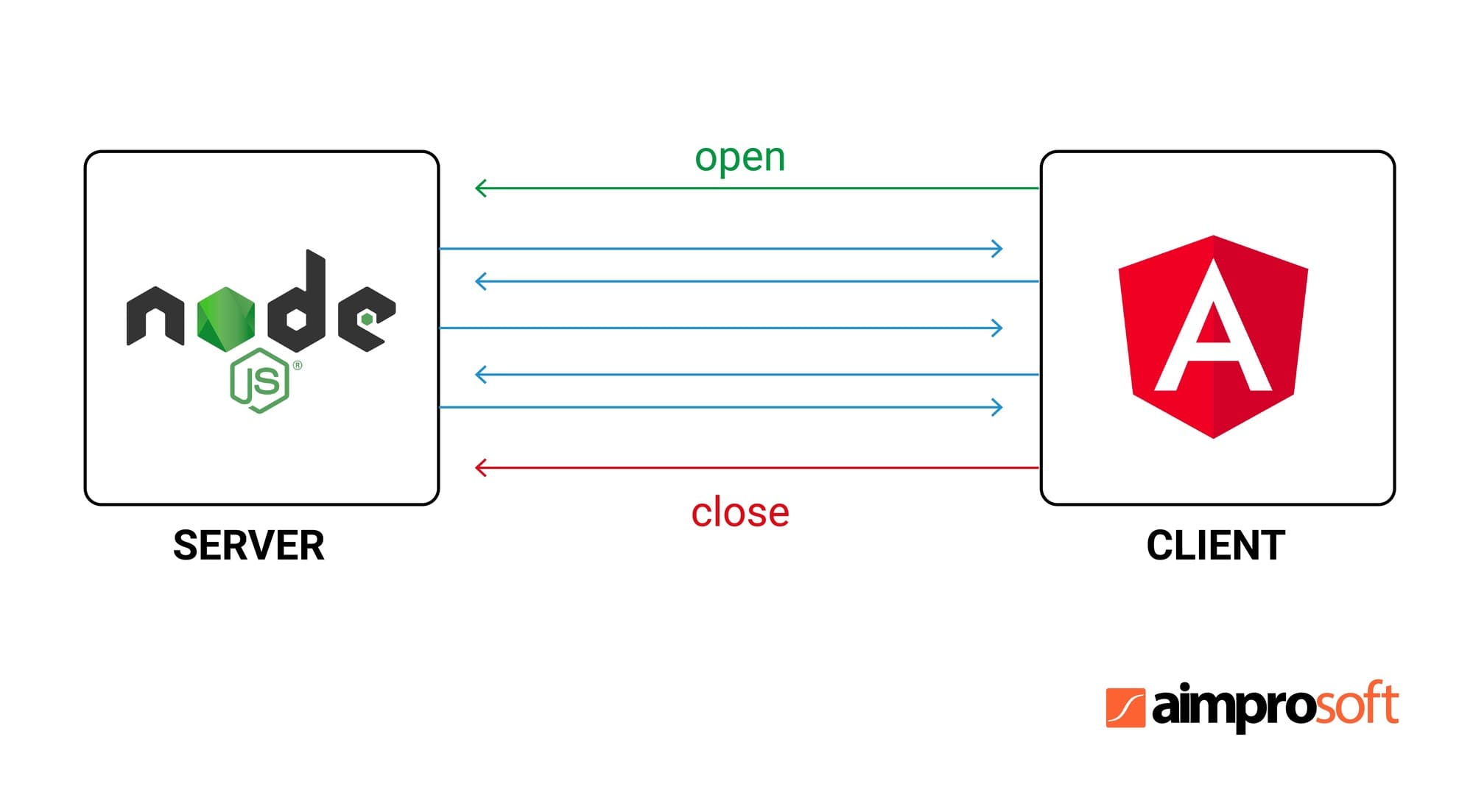
Image: www.aimprosoft.com
Implementing WebSockets in JavaScript
To implement WebSockets in JavaScript, you can leverage the WebSocket API, which is supported by all major browsers. The basic steps involve:
- Create a new WebSocket object:
var socket = new WebSocket('ws://example.com:8080')
- Handle the open event:
socket.on('open', function()...)
- Send data over the WebSocket:
socket.send('Hello from JavaScript')
- Receive data on the WebSocket:
socket.on('message', function(data)...)
- Handle connection errors:
socket.on('error', function(error)...)
- Close the WebSocket:
socket.close()
Best Practices for Using WebSockets in JavaScript
To maximize the effectiveness of WebSockets, consider the following best practices:
- Use Binary Data: Optimize data transfer speeds by using binary data instead of text messages.
- Handle Timeouts: Set appropriate timeouts to handle connection issues and recover gracefully.
- Consider Server-Sent Events: For unidirectional data flow, consider using Server-Sent Events (SSEs) instead of WebSockets.
- Secure the WebSocket Connection: Implement appropriate security protocols, such as TLS, to secure the communication channel.
- Monitor and Log: Regularly monitor WebSocket connections to identify performance issues or errors.
Websockets In Js
Conclusion
WebSockets are an indispensable tool in the arsenal of JavaScript developers, enabling real-time communication and enhanced user experiences. By harnessing the power of open and persistent connections, WebSockets open up a realm of possibilities in various application domains. By following best practices and leveraging the WebSocket API, developers can create robust and performant applications that seamlessly connect clients and servers.